Rylah's Study & Daily Life
12. JSP - Cookie, Session 본문
1. 쿠키(Cookie)
먹는 쿠키가 아니고 HTTP 프로토콜에 대한 쿠키이다.
HTTP 프로토콜은 상태가 없다. 상태를 기억하지 않는다는 말이다. 웹 브라우저에 대한 응답을 하고 나면 해당 클라이언트와의 연결을 지속하지 않는다.
HTTP 프로토콜은 상태에 대한 지속적인 연결 또한 존재하지 않는다. 그래서 이러한 부분을 해결하기 위해 웹서버에서 웹 브라우저의 정보를 저장한다. 이후에는 웹 브라우저 요청에 포함되어 있는 정보와 서버에 저장되어 있는 각각의 정보를 비교해서 동일한 브라우저에서 온 요청인지 판단한다.
쿠키는 상태가 없는 프로토콜을 위해 상태를 지속시키기 위한 방법이다.
쿠키는 웹브라우저의 정보를 웹 브라우저(클라이언트)에 저장하므로 이후에 서버로 전송되는 요청은 쿠키가 가지고 있는 정보가 같이 포함되서 전송된다. 이 때 웹 서버는 쿠키가 포함되어 있는지 읽어서 판단하게 된다.
웹 브라우저에서 쿠키가 저장되어 접속한 사용자의 정보가 유지되는 것이 이러한 이유이다.
쿠키는 웹 사이트를 접속할 때 생성되는 정보를 담은 임시파일이다. 쿠키는 보통 4kb 이하의 크기로 생성된다.
보통 해당 사이트에 접속할때 아이디와 패스워드를 기억하고 절차 없이 빠르게 접속하기 위함이다.
하지만 개인 정보가 기록되기 때문에 개인 사생활 및 정보 노출에 대한 문제점이 있다. 쿠키를 통한 개인정보 유출 문제가 그것이다.
이를 해소하기 위해 쿠키 거부 기능이 추가 되었고, 쿠키를 거부하게 되면 지속적인 연결을 수행할 수 없다.
쿠키 이론은 이정도로 하고 구체적인 것은 세션에서 알아보기로 하자.
- 쿠키를 사용할 때에는 javax.servlet.http 패키지에 있는 Cookie 클래스를 이용하며, 서블릿에서 만들어져 클라이언트의 브라우저에 의해 저장된다.
- 쿠키 안에는 각각의 브라우저를 판별할 수 있는 정보가 포함되어 있고, 이렇게 쿠키가 클라이언트에 한번 저장이 된 후에 다시 서버로 요청할 떄는 클라이언트에 저장된 쿠키 만의 정보가 요청에 포함되어져 서버로 전송된다.
- Cookie 클래스를 사용한 쿠키 생성
1
|
Cookie cookie = new Cookie(String name, String value);
|
cs |
- 쿠키를 생성한 후에는 반드시 response 객체의 addCookie() 메소드를 사용해서 쿠키를 추가해야 한다.
1
|
response.addCookie(name);
|
cs |
- 쿠키 생성 후 쿠키의 이름에 대응하는 값을 새롭게 지정할 때 setValue() 메소드를 사용한다.
1
|
cookie.setValue(newValue);
|
cs |
쿠키 생성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String cookieName = "id";
Cookie cookie = new Cookie(cookieName, "hongkd");
cookie.setMaxAge(60 * 2);
response.addCookie(cookie);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>쿠키 생성</title>
</head>
<body>
<h2>쿠키를 생성하는 페이지</h2>
"<%=cookieName %>"쿠키가 생성되었습니다. <br>
<form method="post" action="useCookie.jsp">
<input type="submit" value="생성된 쿠키 확인">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>웹 브라우저에 저장된 쿠키 사용하기</title>
</head>
<body>
<h2>웹 브라우저에 저장된 쿠키 사용하기</h2>
<%
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (int i = 0; i < cookies.length; ++i) {
if (cookies[i].getName().equals("id")) {
%>
쿠키의 이름은 "<%=cookies[i].getName() %>"이고
쿠키의 값은 "<%=cookies[i].getValue() %>"입니다.
<%
}
}
}
%>
</body>
</html>
|
cs |
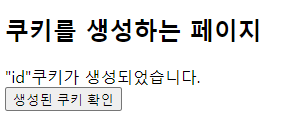
JavaBean
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
package ch12.member;
import java.sql.Timestamp;
public class LogonDataBean {
private String id;
private String passwd;
private String name;
private Timestamp reg_date;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPasswd() {
return passwd;
}
public void setPasswd(String passwd) {
this.passwd = passwd;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Timestamp getReg_date() {
return reg_date;
}
public void setReg_date(Timestamp reg_date) {
this.reg_date = reg_date;
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
|
package ch12.member;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.sql.DataSource;
public class LogonDBBean {
private static LogonDBBean instance = new LogonDBBean();
public static LogonDBBean getInstance() {
return instance;
}
private LogonDBBean() {
}
private Connection getConnection() throws Exception {
Context initCtx = new InitialContext();
Context envCtx = (Context) initCtx.lookup("java:comp/env");
DataSource ds = (DataSource) envCtx.lookup("jdbc/basicjsp");
return ds.getConnection();
}
public void insertMember(LogonDataBean member) throws Exception {
Connection conn = null;
PreparedStatement pstmt = null;
try {
String sql = "insert into member values (?, ?, ?, ?)";
conn = getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, member.getId());
pstmt.setString(2, member.getPasswd());
pstmt.setString(3, member.getName());
pstmt.setTimestamp(4, member.getReg_date());
pstmt.executeUpdate();
} catch (Exception ex) {
ex.printStackTrace();
} finally {
if (pstmt != null)
try { pstmt.close(); } catch (SQLException ex) {}
if (conn != null)
try { conn.close(); } catch (SQLException ex) {}
}
}
public int userCheck(String id, String passwd) throws Exception {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String dbPasswd = "";
int x = -1;
try {
String sql = "select passwd from member where id = ?";
conn = getConnection();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
rs = pstmt.executeQuery();
if (rs.next()) {
dbPasswd = rs.getString("passwd");
if (dbPasswd.equals(passwd)) {
x = 1; // 인증 성공
}
else {
x = 0; // 인증 실패
}
}
else
x = -1; // ID not Found
} catch(Exception ex) {
ex.printStackTrace();
} finally {
if (rs != null)
try { rs.close(); } catch (SQLException ex) {}
if (pstmt != null)
try { pstmt.close(); } catch (SQLException ex) {}
if (conn != null)
try { conn.close(); } catch (SQLException ex) {}
}
return x;
}
}
|
cs |
회원 가입
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>회원가입</title>
</head>
<body>
<h2>회원가입</h2>
<form method="post" action="insertMemberPro.jsp">
아이디 : <input type="text" name="id" maxlength="50"> <br>
패스워드 : <input type="password" name="passwd" maxlength="16"> <br>
이름 : <input type="text" name="name" maxlength="10"> <br>
<input type="submit" value="회원가입">
<input type="reset" value="다시 입력">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<%@page import="ch12.member.LogonDBBean"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%@ page import="ch12.member.LogonDataBean" %>
<% request.setCharacterEncoding("UTF-8"); %>
<jsp:useBean id="member" class="ch12.member.LogonDataBean">
<jsp:setProperty name="member" property="*"/>
</jsp:useBean>
<%
member.setReg_date(new Timestamp(System.currentTimeMillis()));
LogonDBBean logon = LogonDBBean.getInstance();
logon.insertMember(member);
%>
<jsp:getProperty name="member" property="id"/>님 회원가입을 축하합니다.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
</body>
</html>
|
cs |
쿠키 사용 로그인
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>로그인 폼</title>
</head>
<body>
<h2>Login Form Using Cookie</h2>
<form method="post" action="cookieLoginPro.jsp">
아이디 : <input type="text" name="id" maxlength="50"> <br>
패스워드 : <input type="password" name="passwd" maxlength="16"> <br>
<input type="submit" value="로그인">
<input type="button" value="회원가입"
onclick="location.href='insertMemberForm.jsp'">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<%
String id = "";
try {
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (int i = 0; i < cookies.length; ++i) {
if (cookies[i].getName().equals("id"))
id = cookies[i].getValue();
}
if (id.equals(""))
response.sendRedirect("cookieLoginForm.jsp");
} else
response.sendRedirect("cookieLoginForm.jsp");
} catch(Exception ex) {}
%>
<meta charset="UTF-8">
<title>쿠키를 사용한 간단한 회원 인증</title>
</head>
<body>
<b><%=id %></b>님이 로그인 하였습니다.
<form method="post" action="cookieLogout.jsp">
<input type="submit" value="로그아웃">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="ch12.member.LogonDBBean" %>
<% request.setCharacterEncoding("UTF-8"); %>
<!DOCTYPE html>
<html>
<head>
<%
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
LogonDBBean logon = LogonDBBean.getInstance();
int check = logon.userCheck(id, passwd);
if (check == 1)
{
Cookie cookie = new Cookie("id", id);
cookie.setMaxAge(20 * 60);
response.addCookie(cookie);
response.sendRedirect("cookieMain.jsp");
} else if (check == 0) { %>
<script>
alert("비밀번호가 맞지 않습니다.");
history.go(-1);
</script>
<% } else { %>
<script>
alert("아이디가 맞지 않습니다.");
history.go(-1);
</script>
<%} %>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (int i = 0; i < cookies.length; ++i) {
if (cookies[i].getName().equals("id")) {
cookies[i].setMaxAge(0);
response.addCookie(cookies[i]);
}
}
}
%>
<!DOCTYPE html>
<html>
<head>
<script>
alert("로그아웃 되었습니다.");
location.href="cookieMain.jsp";
</script>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
</body>
</html>
|
cs |
결과를 살펴보자.
이런식으로 활용할 수 있다.
2. 세션 (Session)
쿠키가 웹 브라우저에 사용자의 상태를 유지하기 위한 정보를 저장했다면, 세션은 웹서버 쪽의 웹 컨테이너에 상태를 유지하기 위한 정보를 저장하는 것을 말한다.
세션은 사용자의 정보를 유지하기 위해 javax.servlet.http 패키지의 HttpSession 인터페이스를 구현해서 사용한다.
쿠키는 웹 브라우저에 저장된 것을 웹 서버에서 쿠키 정보를 읽어서 사용하는데 이는 웹 서버에서 열어볼 수 있는 것이기에 보안상 문제가 될 수 있다.
세션에서 사용되는 메소드 리스트는 다음과 같다.
Method : Return Type | Description |
getAttribute(java.lang.String name) : java.lang.Object | 세션 속성명이 name인 속성의 값을 Object 타입으로 리턴 속성명이 없을 경우 null 리턴 |
getAttributeNames() : java.util.Enumeration | 세션의 속성의 이름들을 Enumeration 객체 타입으로 리턴 |
getCreationTime() : long | 1970/01/01 0시 0초를 기준으로 세션이 생성된 시간까지 경과한 시간을 계산해서 밀리세컨드 값으로 리턴 |
getId() : java.lang.String | 세션에 할당된 고유 식별자를 String 타입으로 리턴 |
getMaxInactiveInterval() : int | 세션을 유지하기 위해 설정된 세션 유지시간을 int형으로 반환 |
invalidate() : void | 현재 생성된 세션을 무효화 |
removeAttribute(java.lang.String name) : void | 세션 속성명이 name인 속성을 제거한다. |
setAttribute(java.lang.String name, java.lang.Object value) : void | 세션 속성명이 name인 속성에 value를 할당한다. |
setMaxInactiveInterval(int interval) : void | 세션을 유지하기 위한 세션 유지 시간을 초단위로 설정 |
사용자의 정보를 유지하기 위해서는 쿠키보다는 세션을 사용하는 것이 안정적이고 보안상 우위에 있다.
- 서버와 관련된 정보를 노출시키지 않기 위해 쿠키를 사용하는 것 보다 HttpSession 인터페이스의 세션을 통한 상태 관리가 더 효율적이다.
- 세션은 웹 브라우저당 1개씩 생성되어 웹 컨테이너에 저장된다.
- 세션 속성 설정은 session 객체의 setAttribute() 메소드를 사용해서 한다.
1
|
session.setAttribute("id", "goat");
|
cs |
- 세션의 속성을 사용하려면 session 객체의 getAttribute() 메소드를 사용한다.
1
|
String id = (String)session.getAttribute("id");
|
cs |
Object 이므로 사용시 실제 할당된 객체 타입으로 형변환을 해야한다.
- 세션의 속성을 삭제하려면 session 객체의 removeAttribute() 메소드를 사용한다.
1
|
session.removeAttribute("id");
|
cs |
- 세션의 모든 속성을 삭제할 때는 Session 객체의 invalidate() 메소드를 사용한다.
1
|
session.invalidate();
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>세션 테스트</title>
</head>
<body>
<h2>세션 테스트</h2>
<form method="post" action="sessionTestPro.jsp">
아이디 : <input type="text" name="id" maxlength="50"> <br>
패스워드 : <input type="password" name="passwd" maxlength="16"> <br>
<input type="submit" value="정보입력">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>세션 속성 설정 및 사용</title>
</head>
<body>
<%
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
session.setAttribute("id", id);
session.setAttribute("passwd", passwd);
%>
id와 passwd 세션 속성을 설정했습니다.
id 속성의 값은
<%= (String)session.getAttribute("id") %> 이고 <br>
passwd 속성의 값은
<%= (String)session.getAttribute("passwd") %> 입니다.
</body>
</html>
|
cs |
세션 사용 로그인 폼
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h2>Login Form</h2>
<form method="post" action="sessionLoginPro.jsp">
아이디 : <input type="text" name="id" maxlength="50"> <br>
패스워드 : <input type="password" name="passwd" maxlength="16"> <br>
<input type="submit" value="로그인">
<input type="button" value="회원가입"
onclick="location.href='insertMemberForm.jsp'">
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String id = "";
try {
id = (String)session.getAttribute("id");
if (id == null || id.equals(""))
response.sendRedirect("sessionLoginForm.jsp");
else {
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>세션을 활용한 인증</title>
</head>
<body>
<b> <%=id %></b>님이 로그인 하셨습니다.
<form method="post" action="sessionLogout.jsp">
<input type="submit" value="로그아웃">
</form>
</body>
</html>
<%
} catch(Exception ex) {
ex.printStackTrace();
}
%>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="ch12.member.LogonDBBean" %>
<% request.setCharacterEncoding("UTF-8"); %>
<%
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
LogonDBBean logon = LogonDBBean.getInstance();
int check = logon.userCheck(id, passwd);
if (check == 1) {
session.setAttribute("id", id);
response.sendRedirect("sessionMain.jsp");
} else if (check == 0) {
%>
<!DOCTYPE html>
<html>
<head>
<script>
alert("비밀번호가 맞지 않습니다.");
history.go(-1);
</script>
<% } else { %>
<script>
alert("아이디가 맞지 않습니다.");
history.go(-1);
</script>
<% } %>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<% session.invalidate(); %>
<!DOCTYPE html>
<html>
<head>
<script>
alert("로그아웃 되었습니다.");
location.href="sessionMain.jsp";
</script>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
</body>
</html>
|
cs |
새로고침해도 유지 된다,.
'Web Study > JSP' 카테고리의 다른 글
Paging (0) | 2022.03.29 |
---|---|
11. JSP, DataBase (4) - DBCP API (0) | 2022.03.20 |
11. JSP, DataBase (3) - JSP CRUD (0) | 2022.03.20 |
11. JSP, DataBase (2) - Basic Query (0) | 2022.03.20 |
11. JSP, DataBase (1) - MySQL (0) | 2022.03.20 |